Translations
The translation problem
So far in this series we've learnt how to use linear transformation matrices to rotate points around the origin. This is all well and good but it's really not enough to just be able to rotate points, we would also like to translate them. We need to be able to move them around the plane (left/right/up/down etc.) - otherwise we would be stuck designing robots that just spin around on the spot!
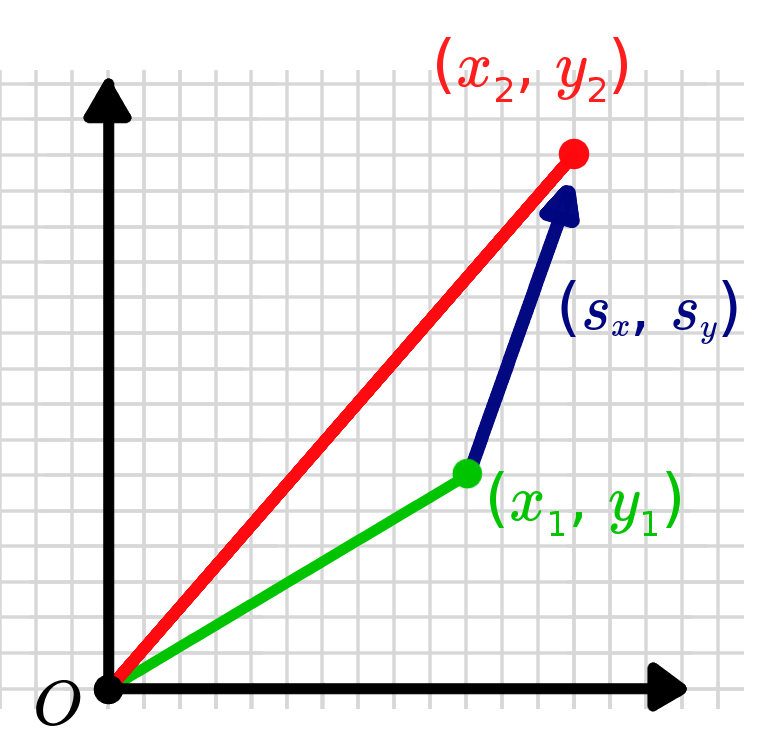
On the surface, this seems like a very straightforward problem to solve - we simply need to add the appropriate amount to the and coordinates. Say, for example, that we had the point and we wanted to shift it by units in the x direction and units in the y direction. We simply perform the following addition:
Seems simple enough, right? The problem is that this operation is non-linear. If you remember, all the transformations we've looked at so far have been of the form which is linear. But our translation looks like .
While it's not the end of the world, having to introduce this nonlinearity is a bit unfortunate. We suddenly lose all those key properties we had with linear transformations, most notably how easily we could chain different transformations together. You'll recall that if we had three nested transformations we could simply multiply the matrices together: .
Let's take a look at what happens if we want to perform the following steps:
- Shift a point by , then
- Rotate it by , then
- Shift it again by , then
- Rotate it by
This chain produces the following equation:
If we were to write these matrices out in full, the equation quickly becomes very confusing. On top of that, inverting the combined transformation becomes really awful. There must be a better way! Thankfully, there is.
Introducing... Homogeneous Coordinates!
To solve this problem we're going to introduce a slightly modified representation of our coordinates. This new system is called homogeneous coordinates. What we'll discover in this post and the next is that by using a homogeneous coordinate system, we can represent both rotations and translations using a single matrix. In this post, we'll focus solely on the translation.
The first thing we have to do is modify our coordinates, which simply involes tacking a "" onto the end of our point vector. For the next little while we will use the bar () above our various variable names to express that they are working with the homogeneous coordinates, but in later posts it will just be assumed.
Deriving the Translation Matrix
What we want to try to do now is to find a linear transformation in this new coordinate system that would represent our translation. In 2D, this will mean we are looking for a matrix to multiply by that is equivalent to adding .